In the dynamic sphere of mobile application development, a perfect blend of creativity, functionality, and seamless user experience drives success. Today, Flutter has emerged as a powerhouse for cross-platform development, enabling developers to write code once and deploy it on multiple platforms—Android, iOS, web, and desktop. As a top flutter app development company specializing in cross-platform app development using Flutter, we understand the importance of robust app testing. But how do you build an effective Flutter mobile app testing strategy? This comprehensive guide will enlighten you.
The Importance of a Solid Testing Strategy
Creating an app goes far beyond just writing code. It involves validating that the code functions as expected under various scenarios, across different devices and operating systems. While the magic of Flutter lies in its "write once, run anywhere" principle, it also presents a unique challenge—ensuring your app provides a consistent, high-quality user experience everywhere.
Imagine this: your app runs flawlessly on an Android device but crashes on iOS, or it looks visually appealing on newer devices but disorganized and unattractive on older ones. These inconsistencies can lead to poor user reviews, lower ratings, decreased trust, and ultimately, a decline in user base. Therefore, a robust testing strategy is not just a 'nice-to-have'; it's a critical necessity.
Benefits of testing in Flutter app development
Testing provides numerous benefits that contribute to the success of your Flutter app:
- Bug Detection: The primary purpose of testing is to identify and fix errors, bugs, and inconsistencies before they reach the end-user. This process helps ensure a smooth user experience and minimizes the likelihood of negative reviews due to technical issues.
- User Trust and Retention: A well-tested app functions reliably, fostering trust among users. This reliability can lead to higher user retention rates, as satisfied users are more likely to continue using an app and recommend it to others.
- Cost and Time Efficiency: Identifying and fixing bugs early in the development process—when they are less costly and easier to resolve—can save substantial time and resources in the long run. A thoroughly tested app also requires fewer updates and patches post-launch, contributing to efficiency.
- Improved Product Quality: Testing enables developers to assess the functionality, usability, and performance of the app, leading to overall improved product quality.
Understanding Flutter Testing Levels
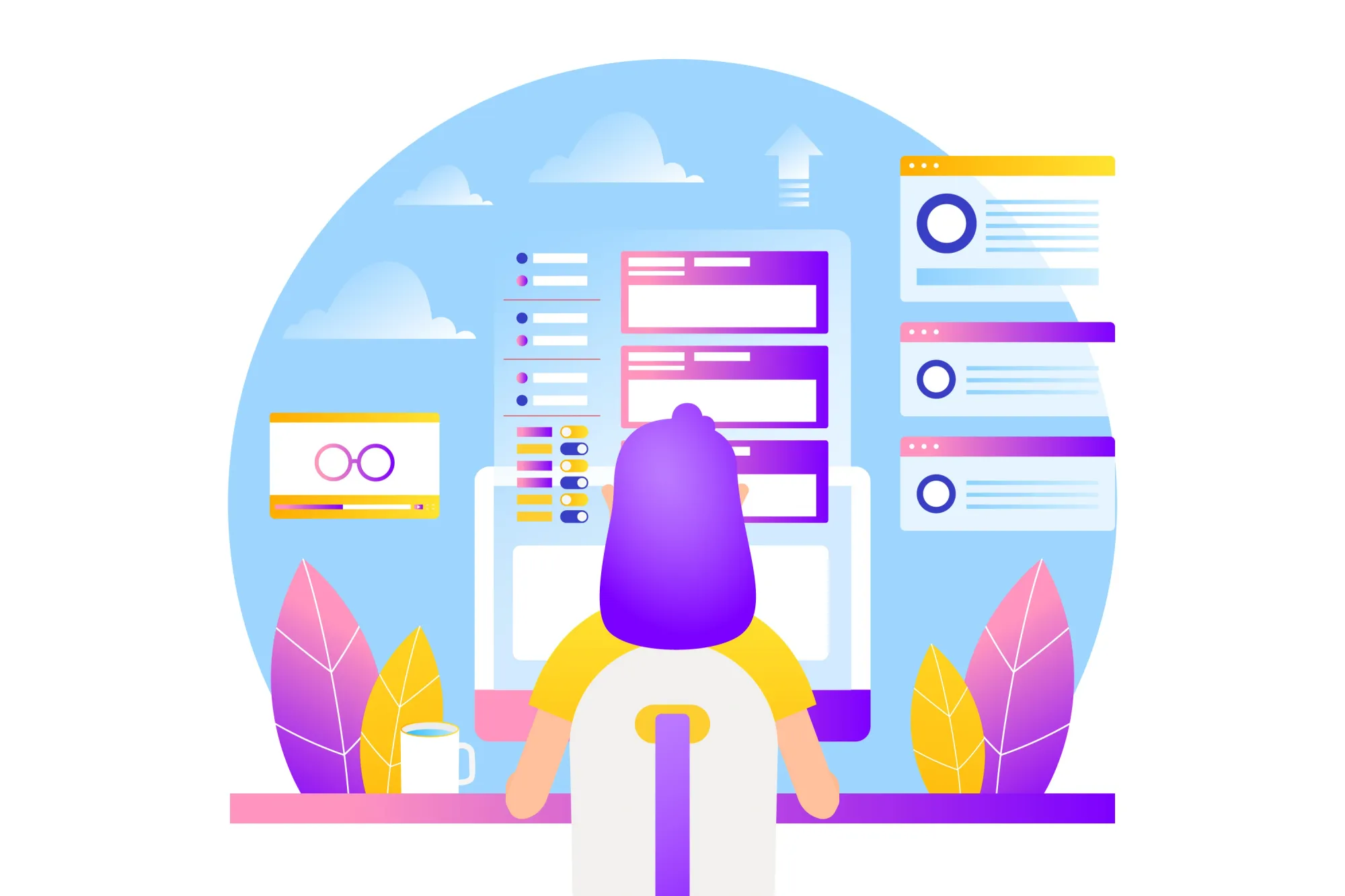
To build an effective testing strategy, understanding the different types of testing is crucial. In Flutter, we primarily focus on three types of tests: unit tests, widget tests, and integration tests. Each type addresses different aspects of your application and collectively, they help validate your app’s behavior at multiple levels.
Unit tests
Unit tests are the foundation of your testing strategy. They validate the functionality of single functions, methods, or classes—essentially, the smallest testable parts of your application. In the context of a shopping app, for example, a unit test might validate whether the 'add to cart' function is working correctly when a user adds an item to their shopping cart.
Unit tests are generally simple, quick to write, and fast to execute, making them ideal for catching issues early in the development process. However, while unit tests are valuable, they cannot catch every issue—particularly those that arise from the interaction between different parts of your app. This is where widget tests come into play.
Widget tests
In Flutter, everything is a widget. A button, a text field, even the app itself is a widget. Widget tests, also known as component tests, verify the functionality of individual widgets in isolation. They ensure that each widget—whether it’s a button, a form, or a screen—behaves as expected.
Consider a login screen on your app, which might include text fields for username and password, a 'submit' button, and a 'forgot password' link. A widget test could verify that when the user fills in their credentials and taps the 'submit' button, the app responds correctly—such as by navigating to the home screen.
Widget tests offer a more comprehensive test scope than unit tests but remain less extensive than integration tests. They are a bit more complex and slower to run than unit tests, but they provide an excellent way to test your app's UI and the interaction of multiple units.
Integration tests
Integration tests, often referred to as end-to-end tests (although these are two different types), assess the application as a whole. They test how various parts of your app work together by simulating real user interactions. Integration tests check full workflows within your app—for example, the process of a user logging in, adding items to their cart, checking out, and receiving a confirmation of their order.
These tests provide the highest level of confidence that your app works correctly but are the most complex to write and slowest to run. However, they're essential for validating user experiences and should be a part of your Flutter app testing strategy.
Key Tools for Flutter Testing
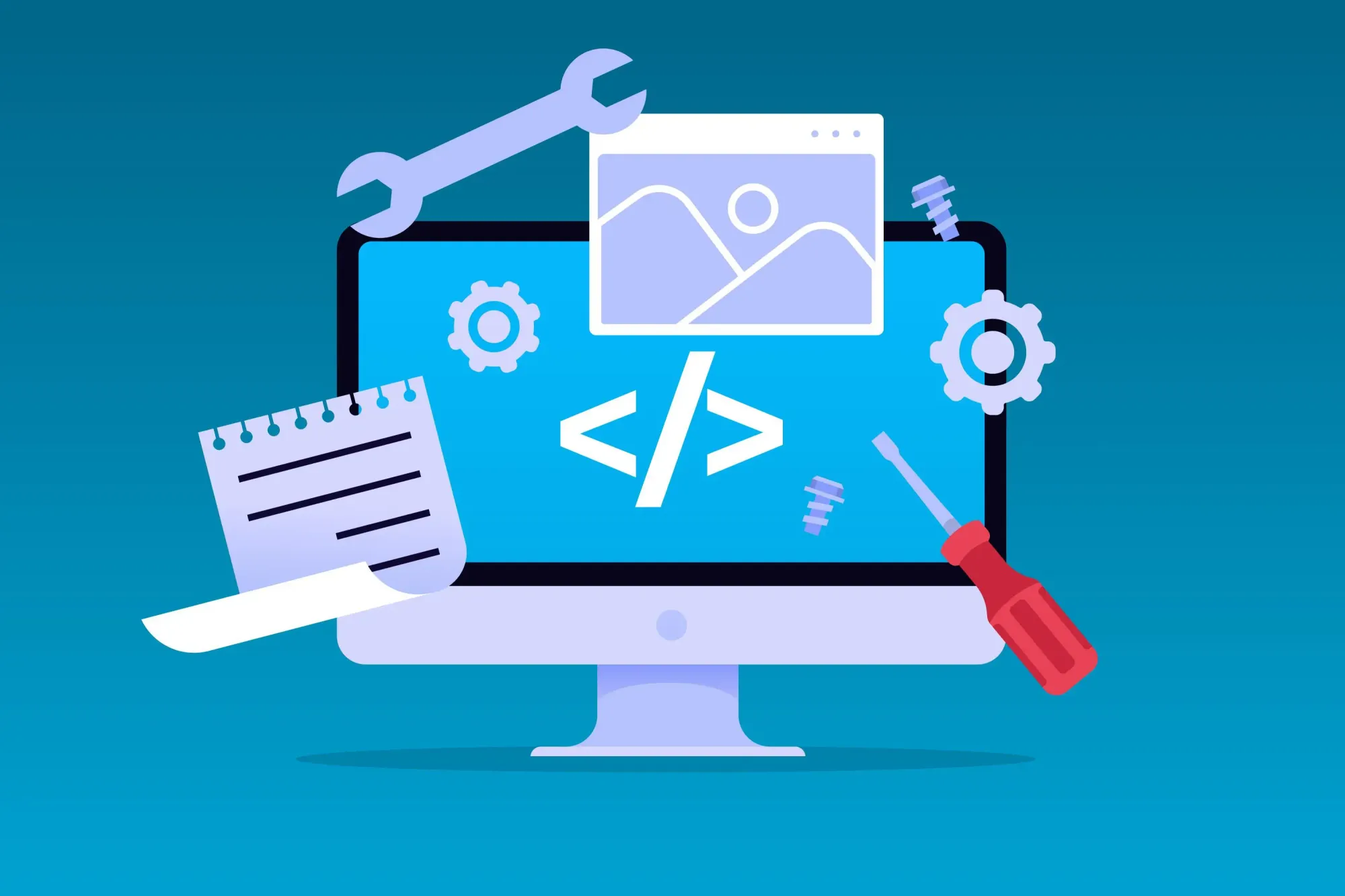
Flutter comes with a rich testing framework that supports unit, widget, and integration tests. However, various other mobile testing tools can complement this framework and streamline your mobile application testing process.
Flutter's testing framework
Flutter's built-in testing library is robust, providing features to facilitate all types of tests. It allows you to create and execute tests right in your IDE, with clear error messages to help you debug any issues.
For unit tests, Flutter provides a rich set of assertions to validate every possible scenario. For widget tests, the framework offers "widget testers" that allow you to build and interact with widgets in a testing environment. Finally, for integration tests, Flutter provides a separate package, flutter_driver
, which lets you create tests that interact with your app just like a real user.
CI/CD tools
Continuous Integration/Continuous Deployment (CI/CD) tools are the backbone of modern application development. They automate the testing process, ensuring that your codebase's health is constantly checked. Tools like Jenkins, CircleCI, or Travis CI, can automatically build your app and run tests every time a change is made, providing quick feedback on any introduced issues.
Let's consider Jenkins, a widely used open-source CI/CD tool. It can be set up to monitor your code repository for changes, automatically trigger a build when changes are detected, run your suite of tests, and then report the results. This automation reduces the chance of human error and significantly decreases the turnaround time for detecting and resolving issues.
Device farm
Flutter enables you to build apps that run on a variety of platforms and devices, but it also means your testing needs to be just as diverse. You should test your app on a wide array of devices with different screen sizes, resolutions, and operating systems to ensure it looks good and functions correctly everywhere.
Manually maintaining such a variety of devices is impractical and expensive. Here, cloud-based device farms like AWS Device Farm or Firebase Test Lab come to the rescue. Firebase Test Lab, a product by Google, allows you to test your apps on a wide variety of devices and configurations hosted in a Google data center. It provides detailed reports to help you understand the performance of your app and identify potential issues. You can access hundreds of device types and configurations to test your app, ensuring it delivers a consistent experience for all users.
Common Testing Challenges
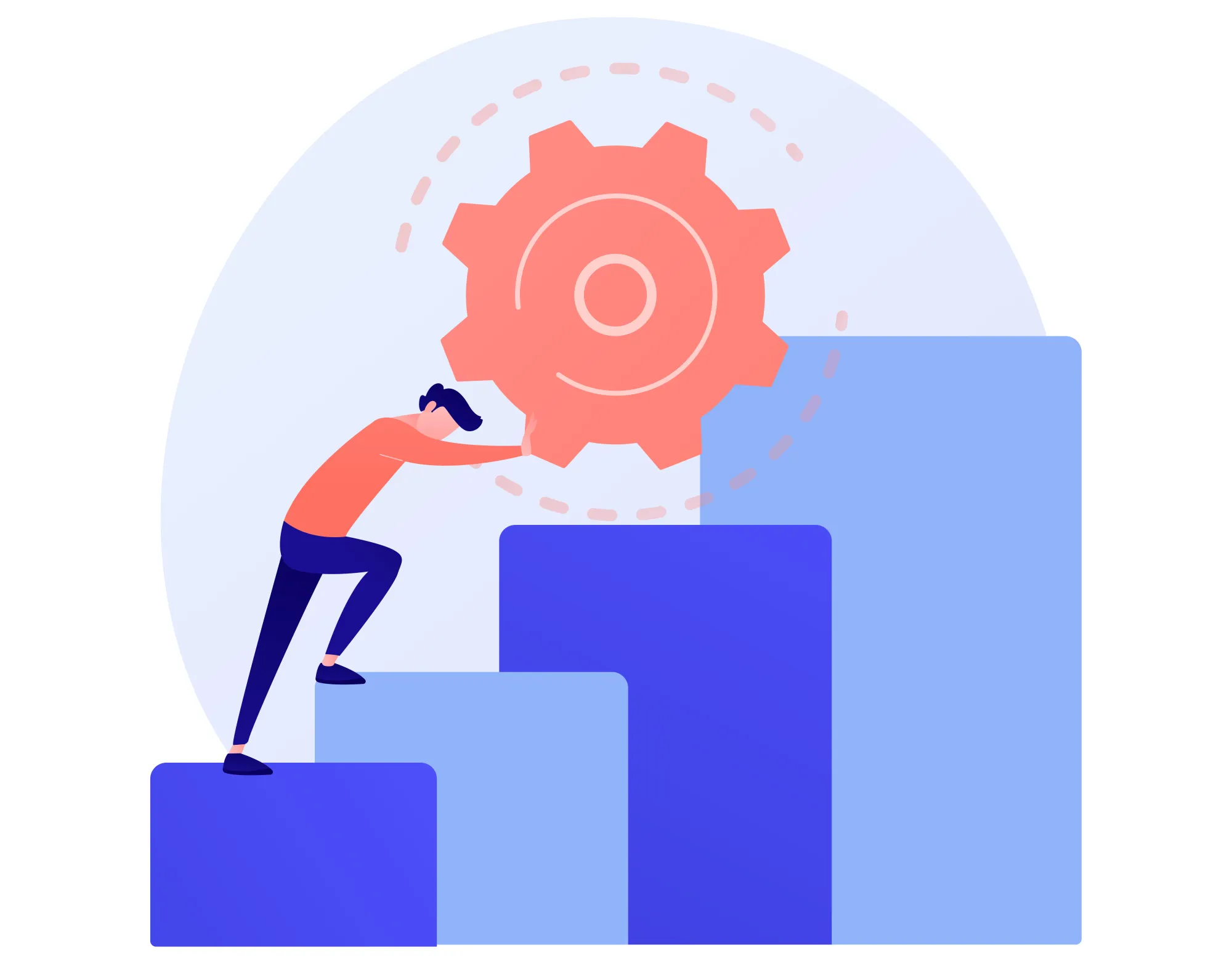
Testing in Flutter, like in any other framework, comes with its unique set of challenges. Here are a few common ones and strategies to tackle them:
Asynchronous code
Flutter makes extensive use of asynchronous operations—for example, making a network request to fetch data. Testing this asynchronous code can be tricky. Flutter's testing framework provides utilities like Future.delayed
and async/await
to make testing asynchronous code easier.
In addition to Futures, Flutter also uses Streams for handling asynchronous operations. Streams are sequences of asynchronous events, and they are especially useful when dealing with continuous incoming data, like user input or a data feed.
Testing Streams can be a bit more complex than testing Futures because you're dealing with a sequence of events over time. However, Flutter's testing framework provides utilities to handle this as well. You can use the StreamMatcher
class to expect certain sequences of events, and the emitsInOrder
function to check that a Stream emits the expected events in the correct order.
Global state management
If your app uses global state management solutions like Provider or Riverpod, testing might seem complicated. However, most of these solutions provide mechanisms to override the global state with local state during testing, making it easier to test widgets in isolation.
Animation testing
Animations can introduce non-determinism into tests—for example, an animation might be at different stages each time a test runs. To address this, Flutter’s testing framework allows you to "pump" frames of animation in a controlled way, ensuring a consistent testing environment.
Best Practices for Flutter Testing
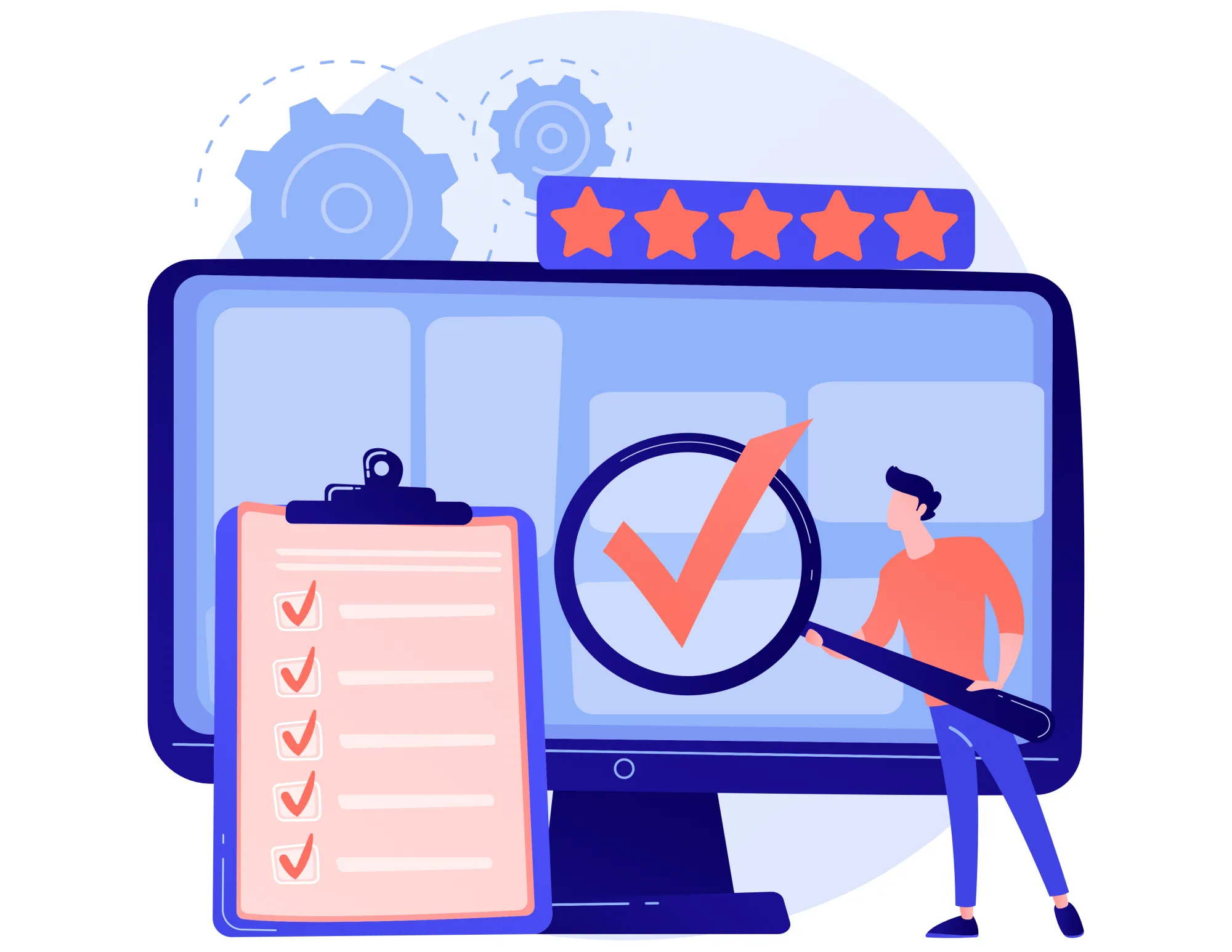
Following best practices can streamline your testing process and make it more effective. Here are a few to consider:
- Adopt test-driven development (TDD): TDD involves writing tests before writing the code itself. It's a powerful approach that ensures every piece of code you write is testable and tested. It encourages simpler designs and inspires confidence.
- Use meaningful test names: A test name should clearly state what functionality or behavior it's testing. Clear test names make it easier to understand what's being tested and to debug issues when a test fails.
- Test all possible cases: strive to test all possible edge cases and scenarios, not just the 'happy path'. For instance, test how your app behaves with no internet connection or when an API call fails.
- Maintain a testing pyramid: your testing strategy should resemble a pyramid: a large base of unit tests (since they are quick to write and run), a smaller number of widget tests, and an even smaller number of integration tests (since they are complex and slow to run).
- Refactor and maintain your tests: just like your app's code, your tests require maintenance. Remove obsolete tests, refactor complex ones, and update tests to reflect changes in your app.
Conclusion
In the realm of Flutter mobile app development, effective testing can make the difference between an app that delights users and one that frustrates them. By building a solid testing strategy that incorporates different types of tests, judicious use of testing tools, and adherence to best practices, you can ensure your Flutter app is ready to impress its audience.
At What the Flutter we have mastered the art of creating delightful, bug-free apps. By leveraging overcoming testing challenges, and adhering to best practices, we cam ensure your Flutter app stands out in today's competitive app marketplace.
Remember, testing is not an afterthought, but an integral part of the development process that must be embedded from the start. With a well-crafted testing strategy, your Flutter app will not only function as intended but also provide a smooth and enjoyable experience for all users.