Introduction
The size of your mobile app’s binary can have a direct impact on its success. Larger app sizes lead to slower downloads, increased data usage, and higher chances of users abandoning the installation process — especially in regions with limited internet access. A bloated binary also eats up valuable device storage, leading to more uninstalls over time.
For businesses, these issues translate to lower user retention rates, poor app performance, and missed revenue opportunities. Reducing the size of your Flutter app not only improves user experience but also enhances your app’s visibility, increases download rates, and contributes to better long-term customer satisfaction.
This guide provides a look at why Flutter app binaries can be large and offers actionable strategies to reduce their size without compromising functionality or user experience. Whether you are developing a new app or maintaining an existing one, these tips will help you optimize your Flutter app for better performance and smaller binaries.
Strategies to Reduce Flutter Binary Size
Optimize image assets
Images are often the biggest offenders when it comes to app size bloat. Here are key strategies to minimize their impact:
- Use vector graphics (SVG): Instead of using raster images (PNG, JPG), consider using SVGs for simple shapes and icons. Vector graphics scale without losing quality and have significantly smaller file sizes.
- Compress images: Tools like TinyPNG or the
flutter_image_compress
package can help compress images without noticeably affecting quality. Compressed images use less storage and reduce download times. - Resize and use proper resolutions: Avoid including high-resolution images if they are not needed. Make sure the images are appropriately scaled for different devices using multiple resolutions (e.g.,
@2x
,@3x
).
Compress fonts
Many apps use custom fonts, which can be large and contain unnecessary characters. Subsetting fonts allows you to include only the characters that your app needs (e.g., specific alphabets or symbols), significantly reducing the font file size.
- Tools like Font Squirrel can help you subset fonts.
- Include only the necessary font weights and styles to reduce size further.
Optimize pubspec.yaml
Third-party packages can add significant overhead to your binary size, especially if they include large libraries or resources. Here’s how you can handle dependencies effectively:
- Audit Assets: Periodically audit your
pubspec.yaml
file and remove any unused images, fonts, or other resources. This ensures that the final binary contains only what is needed. - Audit packages: Check the libraries you are including. If a package is not critical or offers only minor functionality, consider removing it.
- Use lightweight alternatives: In many cases, there are alternative libraries that offer similar functionality but with smaller footprints. For example,
cached_network_image
is a lighter solution for handling images compared to other heavyweight libraries. - Use dependency overriding: Sometimes, third-party packages bring in large dependencies indirectly. You can use Flutter's dependency override mechanism to replace these with more efficient alternatives where appropriate.
Split APKs (for Android)
When targeting Android devices, you can reduce the binary size by generating architecture-specific APKs. Instead of bundling every version of the app (ARM, ARM64, x86) into a single APK, you can split the APK into smaller versions for each architecture. Here’s how to implement this:
- Add the following command to your build process:
flutter build apk --split-per-abi
- This creates separate APKs for each architecture, reducing the size by only including the necessary compiled code for each architecture. For example, an ARM64 APK will only include ARM64 code, making it smaller.
App bundle format (for Android)
Google encourages developers to use Android App Bundles (AAB) instead of APKs for distributing apps. App Bundles optimize the delivery process by splitting the app into various modules and dynamically delivering only the necessary parts for each device. This significantly reduces the download size for users.
- To generate an app bundle, use:
flutter build appbundle
- When published to the Play Store, Google Play automatically manages which resources are delivered to each user based on their device specifications (e.g., architecture, language).
Use Proguard for obfuscation and shrinking (Android)
Proguard is a tool that can be used to obfuscate, shrink, and optimize your app's code. It removes unused code, renames classes and methods, and optimizes bytecode to reduce the final APK size. Proguard is particularly useful when working with large third-party libraries.
- To enable Proguard in your Flutter app, add the following lines to
android/app/build.gradle
under therelease
build type:
buildTypes {
release {
minifyEnabled true
proguardFiles getDefaultProguardFile('proguard-android-optimize.txt'), 'proguard-rules.pro'
}
}
- You can customize Proguard rules in the
proguard-rules.pro
file to ensure that necessary classes and methods are not removed. This can lead to significant reductions in binary size.
Code obfuscation and minification (iOS)
Similar to Proguard for Android, iOS has tools for code minification and obfuscation. You can enable symbol obfuscation in Xcode for release builds, which strips unnecessary symbols and reduces the app's size.
- In Xcode, go to
Build Settings
->Swift Compiler - Code Generation
and enable "Strip Swift Symbols." - Using Flutter's tree-shaking compiler allows automatically exclude unused code in your Dart files.

Remove unused Dart code
Unused Dart code in your project can also contribute to binary size. Use tools like dart pub global run tuneup
to identify and remove unused code. Regularly refactor your code to ensure that you’re not including redundant or dead code in your final build.
Use platform-specific features smartly
Sometimes, developers include plugins that are only necessary for one platform (e.g., a GPS plugin for Android). By configuring the build to exclude platform-specific plugins in the iOS build, you can save space. Make sure to set up platform-specific dependencies in pubspec.yaml
correctly to exclude them when not needed.
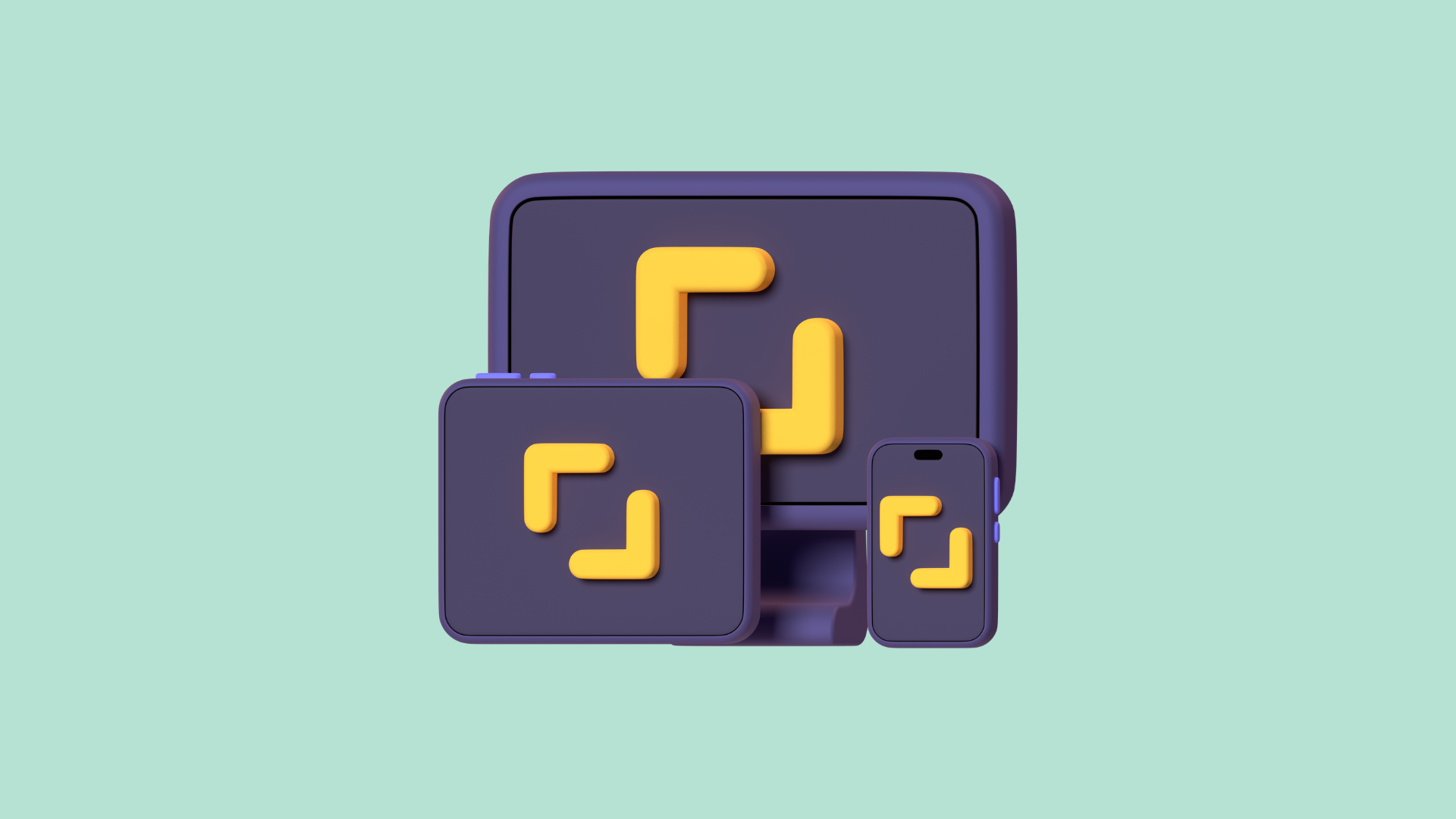
Monitoring and Analyzing Binary Size
One of the most effective ways to manage and reduce your Flutter app's binary size is by consistently monitoring and analyzing its growth throughout the development cycle. Flutter provides built-in tools, such as DevTools, that allow developers to inspect, analyze, and identify which parts of their app contribute the most to the final binary size. By leveraging these tools, you can gain a deeper understanding of what’s inflating your app size and make informed decisions on how to optimize it.
Using Flutter’s build commands for size analysis
Flutter provides tools to help you monitor and analyze your app’s size. These tools can give you insights into which parts of your app are contributing to the size and where optimizations can be made.
- Run the following commands to analyze the APK and iOS build sizes:
flutter build apk --analyze-size
flutter build ios --analyze-size
- The output includes a detailed size breakdown, allowing you to identify areas for improvement. For example, you can see which assets or dependencies are taking up the most space and optimize them accordingly.
Understanding the size analysis report
After running the --analyze-size
command, Flutter generates a JSON file containing a detailed size analysis report. This file can be imported into DevTools for an interactive visualization. Here's how to use it:
- Import the size report: Once in DevTools, you can import the size analysis JSON file generated by your build. Navigate to the Size & Memory tab and load the file to visualize the binary size breakdown.
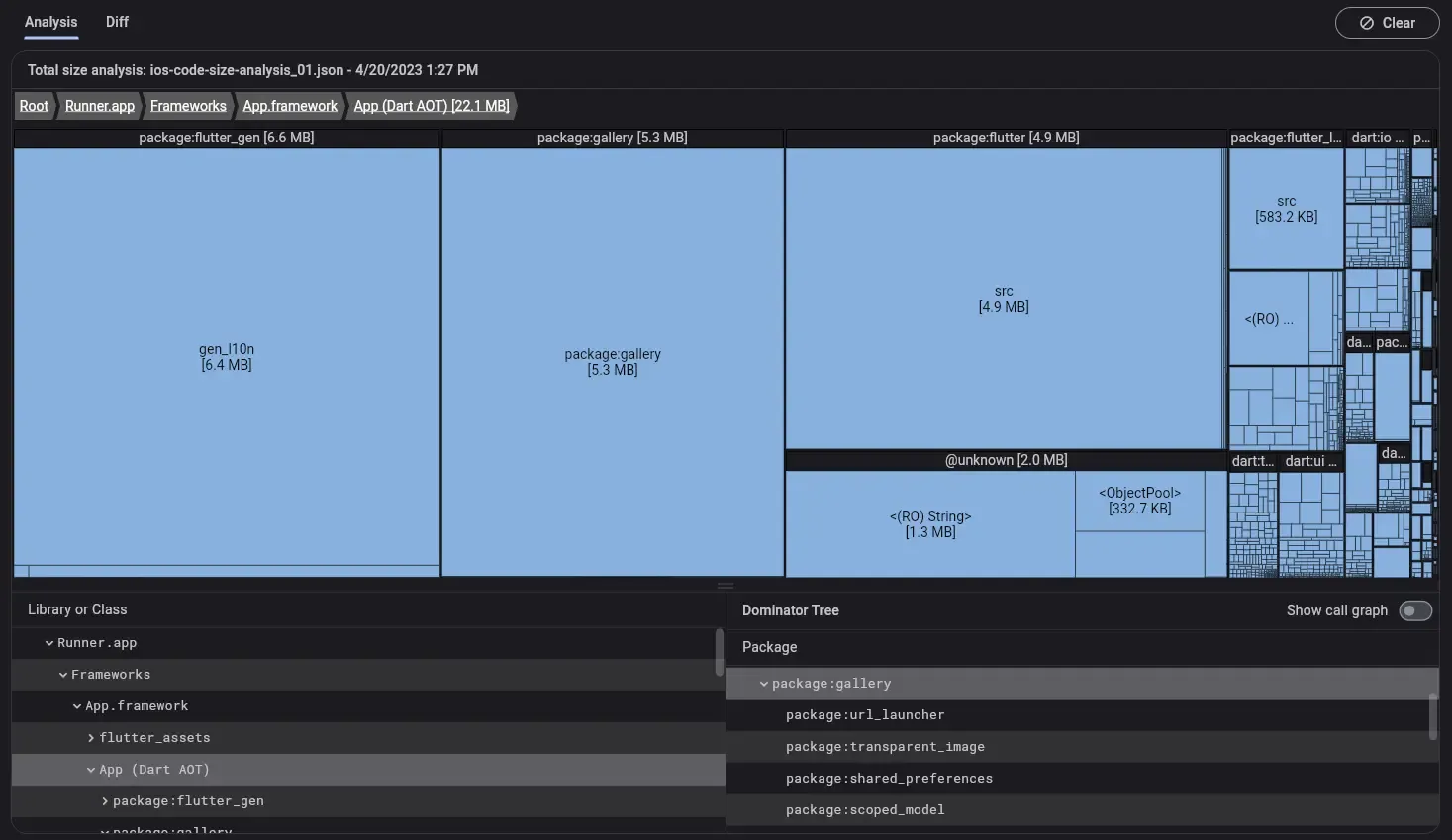
Conclusion
Reducing the binary size of your Flutter app is not just a technical exercise; it’s a necessary step toward delivering a smoother, faster, and more user-friendly experience. A leaner app leads to faster downloads, better performance, and, ultimately, higher user retention, all of which are important for your business success.
At What the Flutter, we specialize in creating optimized, high-performance Flutter apps that delight users and drive engagement. Whether you’re looking to reduce your app’s size, boost performance, or develop a new app from scratch, we’re here to help you every step of the way. Contact us to discuss how we can help make your app as efficient, user-friendly, and competitive as possible!