Introduction
Mobile app performance has to go in tandem to be built seamlessly and correctly. Slow or unresponsive apps cause user dissatisfaction, negative reviews, and user abandonment. So, businesses need to prioritize app performance.
You've already made a fantastic choice by selecting Flutter for your mobile app development. Flutter is renowned for its ability to create beautiful, native-looking apps for both iOS and Android using a single codebase. But a stunning app is only half the battle. A smooth, responsive user experience (UX) is crucial for retaining users and driving business success.
This article will provide you with guidance on best practices to be used when trying to get the most performance out of your Flutter app to keep your business ahead in this highly growing market for mobile apps.
Understanding Flutter Performance
In the context of Flutter apps, performance primarily revolves around two key metrics:
- Rendering speed: This refers to how quickly Flutter can generate the pixels that make up your app's UI on the screen. Ideally, Flutter should be able to render each frame in roughly 16 milliseconds (ms) to achieve a smooth 60 frames per second (FPS) experience. This ensures a seamless and responsive interaction for the user.
- Frame rate (FPS): FPS refers to the number of times per second that the app's UI is updated and redrawn on the screen. A higher FPS translates to a smoother and more fluid user experience. Conversely, a low FPS can lead to choppiness, lag, and a feeling of sluggishness.
Factors affecting Flutter app performance
Several factors can influence the performance of your Flutter app. Here's a breakdown of some key contributors:
- Widget tree complexity: Flutter builds your app's UI using a hierarchy of widgets. A complex widget tree with many nested widgets can take longer to render, impacting performance.
- Widget rebuild frequency: Flutter rebuilds the entire widget subtree whenever a change occurs in its state, even if the change only affects a small portion of the UI. This can be a performance bottleneck for frequently updated widgets or those deeply nested within the widget tree.
- State management strategy: The way you manage your app's state can significantly impact performance. Poor state management practices can trigger unnecessary widget rebuilds, leading to slowdowns.
- UI complexity: Visually complex UIs with rich animations, heavy layouts, or large images can require more processing power to render, potentially affecting performance.
- Device capabilities: The performance of your app will also be influenced by the user's device. Devices with lower processing power, limited memory, or slower network connections will experience slower app performance.
While understanding the theoretical aspects of performance is valuable, it's crucial to have practical tools at your disposal to measure and profile your Flutter app's actual performance. Here's how you can get started:
Measuring performance
Several built-in tools and techniques can help you assess your Flutter app's performance:
- Flutter DevTools: This suite of developer tools provides a wealth of information about your app's performance. Within DevTools, the
Performance view
allows you to:- Analyze frame rate: Monitor the app's FPS in real-time and identify any drops that might indicate performance issues.
- Track build times: See how long it takes for widgets to rebuild during the build phase. This helps pinpoint potential bottlenecks related to complex widget trees or inefficient build methods.
- Visualize the rendering pipeline: Gain insights into the different stages of the rendering pipeline and identify any bottlenecks that might be slowing down the process.
- The Timeline Class: The
Timeline
methods add synchronous events to the timeline. When generating a timeline in Chrome's tracing format, using Timeline generates "Complete" events. Timeline'sstartSync
andfinishSync
can be used explicitly, or implicitly by wrapping a closure intimeSync
.
Timeline.startSync("Doing Something");
doSomething();
Timeline.finishSync();
Or
Timeline.timeSync("Doing Something", () {
doSomething();
});
Profiling performance
Profiling goes beyond just measuring performance metrics. It involves analyzing how your app utilizes resources like CPU, memory, and network bandwidth. This deeper analysis helps you pinpoint the root cause of performance issues.
- Dart observatory: This tool provides detailed insights into your app's memory usage and allocation. You can use it to identify potential memory leaks or excessive memory consumption that might be impacting performance.
- Performance overlay: Flutter DevTools offer a
Performance Overlay
that can be displayed on top of your running app. This overlay provides real-time information about widget rebuilds, frame rate, and build times, allowing you to visualize performance issues directly within the app itself.
WidgetsApp(
showPerformanceOverlay: true,
// Other properties
);
Best Practices to Improve Flutter App Performance
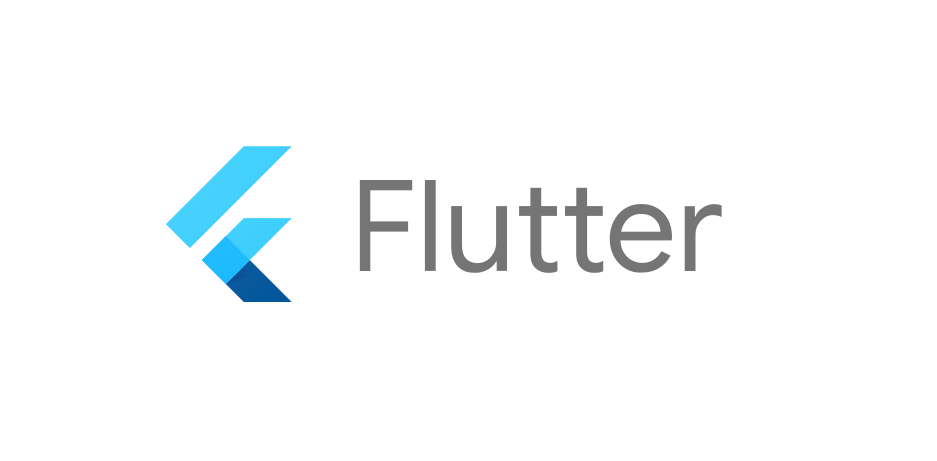
Optimize widget build methods
- Avoid unnecessary widget rebuilds:
- Use
key
properties on widgets that change frequently to prevent unnecessary rebuilds of their entire subtrees. - Consider using
KeepAlive
to preserve the state of widgets that are scrolled off-screen but might reappear soon.
- Use
- Use
const
constructors where possible: By declaring widgets withconst
, you signal to Flutter that the widget is immutable, improving its build performance.
const MyWidget();
- Minimize the use of
StatefulWidgets
: Opt forStatelessWidgets
whenever possible, as they are inherently more performant.
Efficient state management
Efficient state management is crucial for maintaining a high-performance Flutter app. Different state management solutions offer various benefits:
- Use state management solutions like Provider, Riverpod, or Bloc: These packages provide efficient and scalable ways to manage state, reducing unnecessary widget rebuilds and improving app performance.
class MyModel with ChangeNotifier {
// Your state logic
}
- Choose the right state management technique for your app: Depending on your app's complexity and requirements, select a state management approach that fits best. For instance, use Provider for simpler apps and Bloc for more complex state management needs.
Reduce repaints and layouts
Repaints and layouts are resource-intensive operations that can impact app performance. To optimize these processes:
- Avoid large widget trees: Break down complex UIs into smaller, independent widgets. This reduces the number of widgets that need to be rebuilt during a state update.
- Use
RepaintBoundary
to isolate parts of the widget tree: TheRepaintBoundary
widget helps in isolating parts of the widget tree, preventing unnecessary repaints. This approach can significantly reduce the workload on the rendering engine.
RepaintBoundary(
child: MyExpensiveWidget(),
);
- Optimize complex layouts: Explore using
SingleChildScrollView
orGridView
for layouts with long lists or grids, as they are optimized for performance.
Minimize app size
Reducing the size of your app can improve load times and performance.
- Remove unused resources and code: Regularly audit your project to identify and remove any unused images, code, or assets that are bloating your app size. Tools like
flutter analyze
can help with this process. - Use the
--split-per-abi
flag for smaller APK sizes: This flag generates architecture-specific APKs, resulting in a smaller overall download size for users.
flutter build apk --split-per-abi
Leverage asynchronous programming
Asynchronous programming is essential for maintaining a responsive UI.
- Use async/await effectively: Using
async
andawait
allows your app to perform non-blocking operations, keeping the UI responsive.
Future<void> fetchData() async {
final data = await apiService.getData();
// Process data
}
- Avoid blocking the main thread: Ensure that heavy computations and long-running tasks are performed off the main thread to prevent UI freezes.
compute(expensiveFunction, data);
Optimize network calls
Efficient network handling is crucial for app performance.
- Use efficient APIs and data formats: Optimize your APIs and use efficient data formats like JSON to reduce payload sizes and improve response times.
- Implement caching strategies: Implementing caching mechanisms can reduce the number of network requests and improve performance.
class CacheService {
final _cache = <String, dynamic>{};
void cacheData(String key, dynamic data) {
_cache[key] = data;
}
dynamic getData(String key) {
return _cache[key];
}
}
- Reduce the number of network requests: Batching requests and minimizing unnecessary network calls can significantly enhance performance.
Advanced Techniques for Performance Optimization
Custom painting and animations
- Optimize custom painting with
CustomPainter
: UsingCustomPainter
for custom drawing operations can provide better control over rendering performance.
class MyPainter extends CustomPainter {
@override
void paint(Canvas canvas, Size size) {
// Custom painting logic
}
@override
bool shouldRepaint(covariant CustomPainter oldDelegate) {
return false;
}
}
- Use implicit animations where possible: Implicit animations, such as
AnimatedContainer
, are easier to implement and often perform better than explicit animations.
AnimatedContainer(
duration: Duration(seconds: 1),
// Other properties
);
Efficient ListViews
Handling large lists efficiently is critical for performance.
- Use
ListView.builder
for large lists:ListView.builder
is designed for large lists, creating widgets only when they are visible, thus improving performance.
ListView.builder(
itemCount: items.length,
itemBuilder: (context, index) {
return ListTile(
title: Text(items[index]),
);
},
);
- Implement lazy loading and pagination: Implement lazy loading and pagination to load data as needed, reducing initial load times and memory usage.
class PaginatedList extends StatelessWidget {
@override
Widget build(BuildContext context) {
return ListView.builder(
itemCount: items.length,
itemBuilder: (context, index) {
if (index == items.length - 1) {
// Trigger pagination
}
return ListTile(
title: Text(items[index]),
);
},
);
}
}
Image optimization
Efficient image handling can significantly improve app performance.
- Use
cached_network_image
for efficient image loading: Thecached_network_image
package provides efficient image loading and caching, reducing load times and memory usage.
CachedNetworkImage(
imageUrl: 'https://example.com/image.jpg',
placeholder: (context, url) => CircularProgressIndicator(),
errorWidget: (context, url, error) => Icon(Icons.error),
);
- Optimize image sizes and formats: Ensure images are optimized for size and format to reduce load times and improve performance.
Conclusion
By following these best practices and exploring advanced techniques, you can significantly improve the performance of your Flutter app. Remember, performance optimization is an ongoing process. As your app evolves, revisit these strategies and adapt them to your specific needs.
At What the Flutter, we love working with Flutter apps to make them high-performing that delight users. Contact us today to discuss how we can optimize your Flutter app performance.
Frequently Asked Questions (FAQ)
1. What are the key factors that affect Flutter app performance?
Key factors include the efficiency of your code, the use of appropriate widgets, the management of state, the handling of animations and graphics, network calls, and memory usage.
2. What is a good frame rate (FPS) for a Flutter app?
A good target for a smooth user experience is a consistent 60 FPS. Flutter DevTools can help you monitor your app's FPS and identify any drops.
3. How can I tell if my Flutter app is performing well?
Beyond frame rate, look for responsiveness – immediate response to user interactions like taps or scrolls. Use Flutter DevTools and the Performance Overlay to identify potential bottlenecks.
4. Why is my Flutter app slow on some devices?
Device capabilities play a role. Lower-powered devices might struggle with complex UIs or large amounts of data. Consider optimizing your app for a range of devices.
5. Should I always use StatelessWidgets
?
While StatelessWidgets
are generally more performant, use StatefulWidgets
when state management is necessary. Choose the right widget type for the situation.
6. How can I improve the startup time of my Flutter app?
- Defer expensive operations until after the initial build.
- Use lightweight splash screens.
- Minimize the work done in the main() function and during app initialization.
7. What should I do if my app UI is laggy?
- Profile the app using Flutter DevTools to identify the cause of the lag.
- Optimize heavy build methods and animations.
- Reduce the complexity of your widget tree.
8. How can I handle jank during animations?
- Ensure animations are not executed in the build method.
- Use the
AnimationController
andTickerProvider
efficiently. - Profile the animations to find and fix performance issues.
9. What steps can I take if I encounter memory leaks?
- Ensure that all objects, especially controllers and streams, are disposed of correctly.
- Use memory profiling tools to identify leaks.
- Refactor code to use efficient data structures and state management.